コマンドを使ったプラグイン例 開発環境:Xcode7.3.1 Mac OS 10.11.6
これは、キーやジョイスティック等に使われているコマンドをプラグインで利用するということです。
実際はキーやジョイスティックに設定すれば済むことですが、画面にボタンがあれば、常に全面を見ながら操縦が出来るので操作し易いということがあります。プラグイン開発の基本になるスクリプトです。
ここで作成したプラグインはTestEnumsをベースにして作成しています。
この元になっているプラグインは配列を使って、キーコマンドとジョイスティックコマンドの特別コマンド全てを使って作成されています。
今回自作したKeyJoyCommandは、理解しやすいように配列を使わず、単独のコマンドで作成しています。複数のコマンドを作成する場合は同じようなパターンを繰り返して作成するので配列が便利なんですが、最初は把握するのが難解です。配列を使わずにやれば、スクリプトはその分長くなりますが、初心者にとっては非常に理解しやくなります。
ここのポイントは「xplm_key_pause」です。このコマンドでX-Planeをポーズ状態にすることができます。
XPLMCommandKeyStroke(xplm_key_pause);
これがコマンドと紐付けされていて、この名前を入れ変えるだけで他のコマンドを使うこともできます。この他のコマンドはここを参考に。
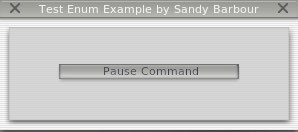
実際はキーやジョイスティックに設定すれば済むことですが、画面にボタンがあれば、常に全面を見ながら操縦が出来るので操作し易いということがあります。プラグイン開発の基本になるスクリプトです。
ここで作成したプラグインはTestEnumsをベースにして作成しています。
この元になっているプラグインは配列を使って、キーコマンドとジョイスティックコマンドの特別コマンド全てを使って作成されています。
今回自作したKeyJoyCommandは、理解しやすいように配列を使わず、単独のコマンドで作成しています。複数のコマンドを作成する場合は同じようなパターンを繰り返して作成するので配列が便利なんですが、最初は把握するのが難解です。配列を使わずにやれば、スクリプトはその分長くなりますが、初心者にとっては非常に理解しやくなります。
ここのポイントは「xplm_key_pause」です。このコマンドでX-Planeをポーズ状態にすることができます。
XPLMCommandKeyStroke(xplm_key_pause);
これがコマンドと紐付けされていて、この名前を入れ変えるだけで他のコマンドを使うこともできます。この他のコマンドはここを参考に。
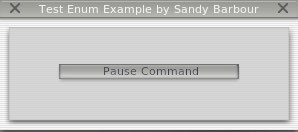
- KeyJoyCommand.zip ダウンロードはこちら
KeyJoyCommand.cpp
/* XPLMCommandKeyStroke Example Written by Sandy Barbour - 23/04/2004 をベースに作成 この例では、キーとジョイスティックコマンドを送信する方法を示しています。 */ #include "XPLMPlugin.h" #include "XPLMUtilities.h" #include "XPLMProcessing.h" #include "XPLMMenus.h" #include "XPLMGraphics.h" #include "XPLMPlanes.h" #include "XPLMDataAccess.h" #include "XPWidgets.h" #include "XPStandardWidgets.h" #include <string.h> #include <stdio.h> #include <stdlib.h> #if IBM #include <windows.h> #endif //------------------------------------------------------------------------- int MenuItem1; //------------------------------------------------------------------------- // ウイジット作成のID XPWidgetID MainWindowWidget = NULL; XPWidgetID SubWindowWidget = NULL; XPWidgetID SendKeyCommandButton = NULL; void TestEnumsMenuHandler(void *, void *); void CreateTestEnumsWidget(int x1, int y1, int w, int h); int TestEnumsHandler( XPWidgetMessage inMessage, XPWidgetID inWidget, long inParam1, long inParam2); //************X-Planeのスタート******************************************************************************** PLUGIN_API int XPluginStart( char * outName, char * outSig, char * outDesc) { //------------------------------------------------------------------------- XPLMMenuID id; int item; //------------------------------------------------------------------------- strcpy(outName, "Key Joy Command"); strcpy(outSig, "xpsdk.examples.KeyJoyCommand"); strcpy(outDesc, "A plug-in that KeyJoyCommand."); //------------------------------------------------------------------------- // メニューの作成 item = XPLMAppendMenuItem(XPLMFindPluginsMenu(), "KeyJoyCommand", NULL, 1); id = XPLMCreateMenu("KeyJoyCommand", XPLMFindPluginsMenu(), item, TestEnumsMenuHandler, NULL); XPLMAppendMenuItem(id, "Key Joy Command", (void *)"KeyJoyCommand", 1); // ウィジェットが表示されているかどうかを知らせるフラグ。 MenuItem1 = 0; return 1; } //------------------------------------------------------------------------- PLUGIN_API void XPluginStop(void) { } //------------------------------------------------------------------------- PLUGIN_API int XPluginEnable(void) { return 1; } //------------------------------------------------------------------------- PLUGIN_API void XPluginDisable(void) { } //------------------------------------------------------------------------- PLUGIN_API void XPluginReceiveMessage(XPLMPluginID inFrom, long inMsg, void * inParam) { } //------------------------------------------------------------------------- // これにより、メニュー選択からウィジェットが作成されます。 // MenuItem1フラグは、それが複数回作成されるのを止めます。 void TestEnumsMenuHandler(void * mRef, void * iRef) { // メニューが選択された場合、ウィジェットダイアログを作成します。 if (!strcmp((char *) iRef, "KeyJoyCommand")) { if (MenuItem1 == 0) { CreateTestEnumsWidget(100, 250, 300, 130); MenuItem1 = 1; } } } //------------------------------------------------------------------------- //ウィジェットのダイアログが作成されます。 //子ウィジェットは入力パラメータに対して相対的に作成しています。 //これにより、ダイアログの位置付けが容易になります void CreateTestEnumsWidget(int x, int y, int w, int h) { int x2 = x + w; int y2 = y - h; char Buffer[255]; strcpy(Buffer, "KeyJoyCommand"); // メインウィジェットウィンドウを作成する---------------------------------- MainWindowWidget = XPCreateWidget(x, y, x2, y2, 1, // Visible Buffer, // desc 1, // root NULL, // no container xpWidgetClass_MainWindow); XPSetWidgetProperty(MainWindowWidget, xpProperty_MainWindowHasCloseBoxes, 1); // サブウィジェットウィンドウを作成する---------------------------------- SubWindowWidget = XPCreateWidget(x+10, y-25, x2-10, y2+10, 1, // Visible "", // desc 0, // root MainWindowWidget, xpWidgetClass_SubWindow); XPSetWidgetProperty(SubWindowWidget, xpProperty_SubWindowType, xpSubWindowStyle_SubWindow); //-------ボタン------------------- SendKeyCommandButton = XPCreateWidget(x+60, y-60, x+240, y-82, 1, " Pause Command", 0, MainWindowWidget, xpWidgetClass_Button); XPSetWidgetProperty(SendKeyCommandButton, xpProperty_ButtonType, xpPushButton); //------------------------------------------------------------------------- // ウィジェットハンドラを登録する XPAddWidgetCallback(MainWindowWidget, TestEnumsHandler); } //------------------------------------------------------------------------- // これはウィジェットのハンドラです。ボタンの押下などを処理するために使用します。 int TestEnumsHandler( XPWidgetMessage inMessage, XPWidgetID inWidget, long inParam1, long inParam2) { void *Param; Param = 0; char Buffer[255]; bool State; //------------------------------------------------------------------------- // 閉じるボタンはメインウィジェット(子ウィジェット)を取り除きます。 if (inMessage == xpMessage_CloseButtonPushed) { if (MenuItem1 == 1) { XPDestroyWidget(MainWindowWidget, 1); MenuItem1 = 0; } return 1; } //------------------------------------------------------------------------- //任意のボタンのプッシュを処理する(ボタンを押したらポーズ) if (inMessage == xpMsg_PushButtonPressed) { if (inParam1 == (long)SendKeyCommandButton) { XPLMCommandKeyStroke(xplm_key_pause); //コマンドキーIDを入れる場合 //XPLMCommandButtonPress(xplm_joy_pause); //ジョイスティックボタンIDを入れる場合 //このコマンドはキー用とジョイスティック用のコマンドどちらでも使えます。 return 1; } } return 0; }