「p」タグと「RichText」のテキストと背景の色を変更する単純なブロックを「create-block」で作成。
編集できない<p>タグだけのテキストと背景のカラー設定
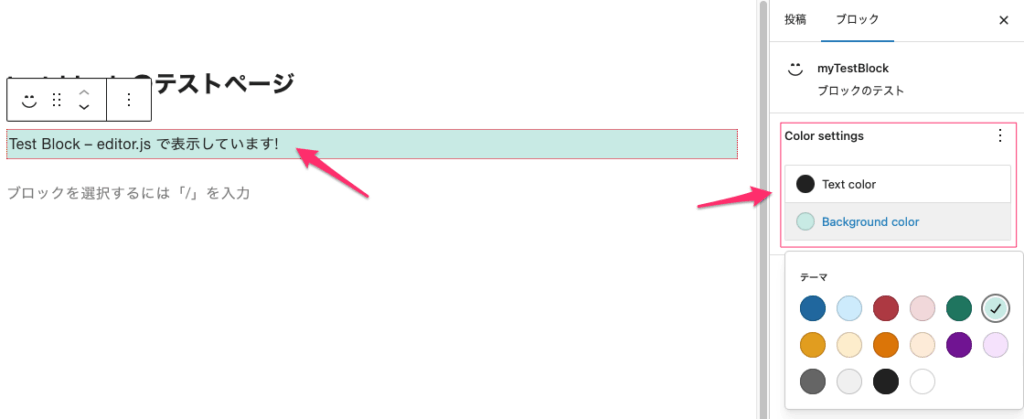
block.jsonはデフォルトにハイライト部分を追加している。
{
"$schema": "https://schemas.wp.org/trunk/block.json",
"apiVersion": 3,
"name": "create-block/test-block",
"version": "0.1.0",
"title": "myTestBlock",
"category": "widgets",
"icon": "smiley",
"description": "ブロックのテスト",
"example": {},
"supports": {
"html": false
},
"attributes": {
"content": {
"type": "string",
"source": "html",
"selector": "p"
},
"backgroundColor": {
"type": "string"
},
"textColor": {
"type": "string"
}
},
"textdomain": "test-block",
"editorScript": "file:./index.js",
"editorStyle": "file:./index.css",
"style": "file:./style-index.css",
"viewScript": "file:./view.js"
}
edit.jsはデフォルトにハイライト部分を変更又は追加している。
import { __ } from '@wordpress/i18n';
import {
useBlockProps, InspectorControls, PanelColorSettings
} from '@wordpress/block-editor';
import './editor.scss';
export default function Edit({ attributes, setAttributes }) {
const blockProps = useBlockProps();
const { backgroundColor, textColor } = attributes;
const onChangeContent = (newContent) => {
setAttributes({ content: newContent })
}
const onChangeBackgroundColor = (newBackgroundColor) => {
setAttributes({ backgroundColor: newBackgroundColor })
}
const onChangeTextColor = (newTextColor) => {
setAttributes({ textColor: newTextColor })
}
return (
<>
<InspectorControls>
<PanelColorSettings
title={__('Color settings', 'test-block')}
initialOpen={false}
colorSettings={[
{
value: textColor,
onChange: onChangeTextColor,
label: __('Text color', 'test-block')
},
{
value: backgroundColor,
onChange: onChangeBackgroundColor,
label: __('Background color', 'test-block')
}
]}
/>
</InspectorControls>
<p {...useBlockProps()}
tagName="p"
onChange={onChangeContent}
style={{
backgroundColor: backgroundColor,
color: textColor
}}
>
{__('Test Block – editor.js で表示しています!', 'test-block')}
</p>
</>
);
}
save.jsはデフォルトにハイライト部分を変更又は追加している。
import { useBlockProps } from '@wordpress/block-editor';
export default function save({attributes}) {
const { backgroundColor, textColor } = attributes;
const onChangeContent = (newContent) => {
setAttributes({ content: newContent })
}
return (
<p {...useBlockProps.save()}
tagName="p"
onChange={onChangeContent}
style={{
backgroundColor: backgroundColor,
color: textColor
}}
>
{'Test Block – save.js で表示しています!'}
</p>
);
}
編集できる<RichText>でのテキストと背景のカラー設定
block.json は上と同じ
{
"$schema": "https://schemas.wp.org/trunk/block.json",
"apiVersion": 3,
"name": "create-block/test-block",
"version": "0.1.0",
"title": "myTestBlock",
"category": "widgets",
"icon": "smiley",
"description": "ブロックのテスト",
"example": {},
"supports": {
"html": false
},
"attributes": {
"content": {
"type": "string",
"source": "html",
"selector": "p"
},
"backgroundColor": {
"type": "string"
},
"textColor": {
"type": "string"
}
},
"textdomain": "test-block",
"editorScript": "file:./index.js",
"editorStyle": "file:./index.css",
"style": "file:./style-index.css",
"viewScript": "file:./view.js"
}
import { __ } from '@wordpress/i18n';
import {
useBlockProps,
RichText,
InspectorControls,
PanelColorSettings
} from '@wordpress/block-editor';
import './editor.scss';
export default function Edit({ attributes, setAttributes }) {
const blockProps = useBlockProps();
const { content, backgroundColor, textColor } = attributes;
const onChangeContent = (newContent) => {
setAttributes({ content: newContent })
}
const onChangeBackgroundColor = (newBackgroundColor) => {
setAttributes({ backgroundColor: newBackgroundColor })
}
const onChangeTextColor = (newTextColor) => {
setAttributes({ textColor: newTextColor })
}
return (
<>
<InspectorControls>
<PanelColorSettings
title={__('Color settings', 'test-block')}
initialOpen={false}
colorSettings={[
{
value: textColor,
onChange: onChangeTextColor,
label: __('Text color', 'test-block')
},
{
value: backgroundColor,
onChange: onChangeBackgroundColor,
label: __('Background color', 'test-block')
}
]}
/>
</InspectorControls>
<RichText
{...blockProps}
tagName="p"
onChange={onChangeContent}
allowedFormats={['core/bold', 'core/italic']}
value={content}
placeholder={__('リッチテキストなので記述できます...')}
style={{
backgroundColor: backgroundColor,
color: textColor
}}
/>
</>
);
}
import { useBlockProps, RichText } from '@wordpress/block-editor';
export default function save({attributes}) {
const blockProps = useBlockProps.save();
const { content, backgroundColor, textColor } = attributes;
return (
<RichText.Content
{ ...blockProps }
tagName="p"
value={ content }
style={ { backgroundColor: backgroundColor, color: textColor } }
/>
);
}