R.id.xxxは定数ではありません。
古いAndroid SDKのコードではswitchのcase文でR.id.xxxを使うことが可能でしたが、途中でR.id.xxxが定数ではなくなったため、最新の環境では使えなくなっています。
しかし、if else if文で書き換えると同じ動作を実現できるようになる。
以下のような、switch文が使えなくなっている。
//ボタンを押されたときの動作を定義するクラスを作成 private class ButtonClickListener implements View.OnClickListener{ //ボタンを押されたときの動作を定義 @Override public void onClick(View view){ //結果を出力するテキストビューのid TextView ChangeColor =findViewById(R.id.color_text); //押されたボタンのidを取得 int id = view.getId(); //押されたボタンのidによって条件分岐 switch (id) { case R.id.button_red://赤色のボタンが押された時の処理 ChangeColor.setText(R.string.red); ChangeColor.setTextColor(Color.RED); break; case R.id.button_blue:// 青色のボタンが押された時の処理 ChangeColor.setText(R.string.blue); ChangeColor.setTextColor(Color.BLUE); break; case R.id.button_yellow:// 黄緑色のボタンが押された時の処理 ChangeColor.setText(R.string.green); ChangeColor.setTextColor(Color.GREEN); break; } } }
if文にすると同じ動作が実現する。しかし実際に動作させるには「onCreate」内にも追加しないといけないものがあるので、この下にある「MainActivity.java」を参考にすること。
//ボタンを押されたときの動作を定義するクラスを作成 private class ButtonClickListener implements View.OnClickListener{ //ボタンを押されたときの動作を定義 @Override public void onClick(View view){ //結果を出力するテキストビューのid TextView ChangeColor =findViewById(R.id.color_text); //赤色のボタンが押された時の処理 if(view.getId() == R.id.button_red) { ChangeColor.setText(R.string.red);//テキストを『赤色』と表示 ChangeColor.setTextColor(Color.RED);//上のテキストを赤色にする // 青色のボタンが押された時の処理 }else if(view.getId() == R.id.button_blue) { ChangeColor.setText(R.string.blue); ChangeColor.setTextColor(Color.BLUE); // オレンジ色のボタンが押された時の処理 }else{ ChangeColor.setText(R.string.green); ChangeColor.setTextColor(color.GREEN); } } }
参考までにこの分のレイアウトとjavaを載せておきます。
プrジェクト名は:SampleColorButton
参考にさせてもらいました:https://zerokara-app.com/299/
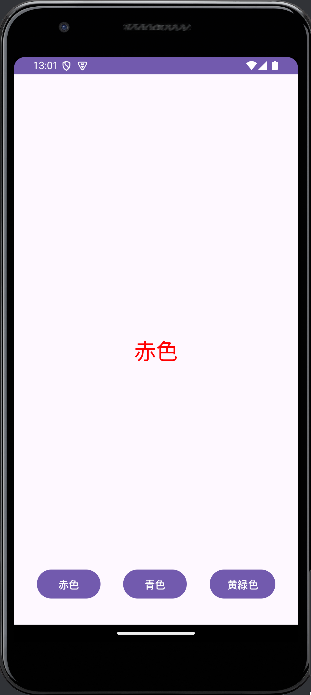
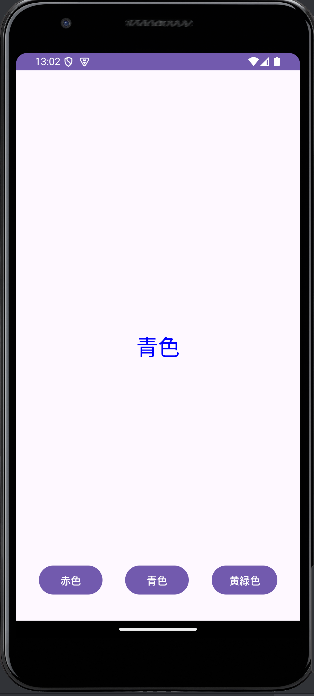
ここには黄色が入る予定だったが色が見えにくいので入れていない。
<resources> <string name="app_name">カラーボタン</string> <string name="red">赤色</string> <string name="blue">青色</string> <string name="yellow">黄緑色</string> <string name="text">ボタンを押してください</string> </resources>
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <TextView android:id="@+id/color_text" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/text" android:textSize="30sp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toRightOf="parent" app:layout_constraintTop_toTopOf="parent" /> <Button android:id="@+id/button_red" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginBottom="32dp" android:text="@string/red" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toStartOf="@+id/button_blue" app:layout_constraintHorizontal_bias="0.5" app:layout_constraintStart_toStartOf="parent" /> <Button android:id="@+id/button_blue" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginBottom="32dp" android:text="@string/blue" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toStartOf="@+id/button_yellow" app:layout_constraintHorizontal_bias="0.5" app:layout_constraintStart_toEndOf="@+id/button_red" /> <Button android:id="@+id/button_yellow" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginBottom="32dp" android:text="@string/yellow" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="0.5" app:layout_constraintStart_toEndOf="@+id/button_blue" /> </androidx.constraintlayout.widget.ConstraintLayout>
package com.example.samplecolorbutton; import androidx.appcompat.app.AppCompatActivity; import android.graphics.Color; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.TextView; public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); //ボタンビューを3つ取得 Button buttonRed = findViewById(R.id.button_red); Button buttonBlue = findViewById(R.id.button_blue); Button buttonYellow = findViewById(R.id.button_yellow); //ボタンを押されたときの動作を持つインスタンスを生成 ButtonClickListener listener = new ButtonClickListener(); //ボタンにButtonClickListenerインスタンスをセット buttonRed.setOnClickListener(listener); buttonBlue.setOnClickListener(listener); buttonYellow.setOnClickListener(listener); } //ボタンを押されたときの動作を定義するクラスを作成 private class ButtonClickListener implements View.OnClickListener{ //ボタンを押されたときの動作を定義 @Override public void onClick(View view){ //結果を出力するテキストビューのid TextView ChangeColor =findViewById(R.id.color_text); //赤色のボタンが押された時の処理 if(view.getId() == R.id.button_red) { ChangeColor.setText(R.string.red);//テキストを『赤色』と表示 ChangeColor.setTextColor(Color.RED);//上のテキストを赤色にする // 青色のボタンが押された時の処理 }else if(view.getId() == R.id.button_blue) { ChangeColor.setText(R.string.blue); ChangeColor.setTextColor(Color.BLUE); // オレンジ色のボタンが押された時の処理 }else{ ChangeColor.setText(R.string.yellow); ChangeColor.setTextColor(Color.YELLOW); } } } }