位置の設定の種類
この位置の設定には色んなパターンがあり、混乱する。
X-Planeの画面に直接表示する場合、フローティングウインドウに表示する場合、それぞれスクリプトの書き方が違う。
目次
単純なX-Planeの画面に表示する場合のクリック
X-Plane上のどこかでマウスクリック、ドラッグするとdatarefの数値を上げることができる。
整数でもfloatでも同じようにできる。

local heading = dataref_table("sim/cockpit2/switches/panel_brightness_ratio") function testDraw() draw_string(400, 400, "Brightness = " .. tostring(heading[0]) ) --値の出力、tostringはデータを文字列として表示するために必要 end do_every_draw("testDraw()") function testClick() if MOUSE_STATUS == "down" then --マウスをdownしたら実行 heading[0] = heading[0] + 0.01 end if MOUSE_STATUS == "drag" then --マウスをdragしたら実行 heading[0] = heading[0] + 0.01 end --dataref数値の制限 if heading[0] < 0 then heading[0] = 0 end if heading[0] > 1 then heading[0] = 1 end if MOUSE_X < 400 then RESUME_MOUSE_CLICK = true end end do_on_mouse_click("testClick()")
単純なX-Planeの画面に表示する場合のクリック。BAROだけをとりだしたもの。
require "graphics" local qs_baro = dataref_table("sim/cockpit/misc/barometer_setting") local qs_real_baro = dataref_table("sim/weather/barometer_sealevel_inhg") function draw_quick_settings() -- グラフィックシステムを初期化する XPLMSetGraphicsState(0,0,0,1,1,0,0) -- 透明な背景を描く graphics.set_color(0, 0, 0, 0.5) --長方形の色(黒)、透明度 graphics.draw_rectangle(100, 70, 600, 100) --長方形を描く graphics.set_color(1, 1, 1, 0.5) --線の色 -- 情報テキストを描く draw_string_Helvetica_10(115, 89, "BARO") --1013.2 ヘクトパスカル(hPa)= 29.92 水銀柱インチ(inHg) --下はdatarefの水銀柱インチ値をヘクトパスカル値に変換している draw_string_Helvetica_18(115, 74, math.floor(qs_baro[0] * 33.8637526 + 0.5))--math.floor=小数点以下切り捨て end do_every_draw("draw_quick_settings()") -- マウスをクリックしたときの動作を設定 function qs_mouse_click_events() -- これは一度だけ反応します if MOUSE_STATUS ~= "down" then return end -- BARO if MOUSE_X > 110 and MOUSE_X < 160 and MOUSE_Y > 70 and MOUSE_Y < 100 then qs_baro[0] = qs_real_baro[0] --クリックでqs_baroの値をqs_real_baroの値にする。 --qs_real_baro値は変換されていないように見えるが、上のqs_baroの戻って変換される。 RESUME_MOUSE_CLICK = true end end do_on_mouse_click("qs_mouse_click_events()") -- マウスホイールをクリックしたときの動作を設定 function qs_mouse_wheel_events() -- BARO if MOUSE_X > 110 and MOUSE_X < 160 and MOUSE_Y > 70 and MOUSE_Y < 100 then qs_baro[0] = qs_baro[0] + MOUSE_WHEEL_CLICKS * 0.01 --1x0.01分、細かく動作させる RESUME_MOUSE_WHEEL = true end end do_on_mouse_wheel("qs_mouse_wheel_events()")
HDGだけをとりだしたもの。
require "graphics" local qs_heading = dataref_table("sim/cockpit/autopilot/heading") local qs_real_heading = dataref_table("sim/flightmodel/position/magpsi") function draw_quick_settings() -- グラフィックシステムを初期化する XPLMSetGraphicsState(0,0,0,1,1,0,0) -- 透明な背景を描く graphics.set_color(0, 0, 0, 0.5) --長方形の色(黒)、透明度 graphics.draw_rectangle(100, 70, 150, 100) --長方形を描く graphics.set_color(1, 1, 1, 0.5) --文字の色 -- 情報テキストを描く draw_string_Helvetica_10(105, 89, "HDG") --テキスト draw_string_Helvetica_18(105, 74, math.floor(qs_heading[0])) --math.floor = 小数点以下切り捨て end do_every_draw("draw_quick_settings()") -- マウスをクリックしたときの動作を設定 function qs_mouse_click_events() -- 私たちは一度だけ反応します if MOUSE_STATUS ~= "down" then return end -- HDG(マウスが反応するスペースを設定) if MOUSE_X > 100 and MOUSE_X < 150 and MOUSE_Y > 70 and MOUSE_Y < 100 then --この範囲をクリックされたら以下を実行 qs_heading[0] = qs_real_heading[0] --クリックで qs_heading を qs_real_heading に入れ替える RESUME_MOUSE_CLICK = true end end do_on_mouse_click("qs_mouse_click_events()") function qs_mouse_wheel_events() -- HDG if MOUSE_X > 100 and MOUSE_X < 150 and MOUSE_Y > 70 and MOUSE_Y < 100 then qs_heading[0] = 10 * (math.floor(qs_heading[0]/10) + MOUSE_WHEEL_CLICKS) --10ずつカウントする if qs_heading[0] < 0 then --0より小さい場合は qs_heading[0] = 350 --qs_headingを350にする end if qs_heading[0] > 360 then --360より大きい場合は qs_heading[0] = 10 --qs_headingを10にする(実際は10ずつカウントなので0になる) end RESUME_MOUSE_WHEEL = true end end do_on_mouse_wheel("qs_mouse_wheel_events()")
X-Plane画面に表示するだけのマウスホイールアクション
X-Plane上のどこかでマウスホイールを回すと以下の画面が出る。トリムの動作をコントロールできる。
OpenGLで描画している、graphicsでも使える。回転する動作では使えない。
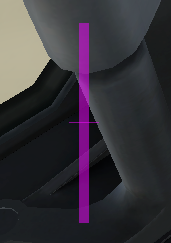
dataref("xp_elv_trim", "sim/flightmodel/controls/elv_trim", "writable") --マウスホイールの動きで移動させる。 function set_trim_by_mouse_wheel() xp_elv_trim = xp_elv_trim - MOUSE_WHEEL_CLICKS * 0.0025 if xp_elv_trim > 1.0 then xp_elv_trim = 1.0 end if xp_elv_trim < -1.0 then xp_elv_trim = -1.0 end RESUME_MOUSE_WHEEL = true end do_on_mouse_wheel("set_trim_by_mouse_wheel()") --スライダーを描画。 function draw_trim_info() XPLMSetGraphicsState(0,0,0,1,1,0,0) glColor4f(1, 1, 1, 0.5) glRectf(100, 100, 110, 300) glBegin_LINES() glVertex2f(90, 200 + xp_elv_trim*100) glVertex2f(120, 200 + xp_elv_trim*100) glEnd() end do_every_draw("draw_trim_info()")
HUDを使ったクリックとマウスホイールアクション
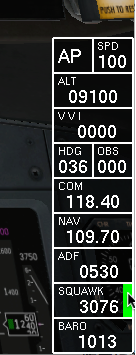
require "graphics" require "HUD" require "radio" require "bit" dataref("ALT", "sim/cockpit2/autopilot/altitude_dial_ft", "writable") dataref("VVI", "sim/cockpit2/autopilot/vvi_dial_fpm", "writable") dataref("ALT_ARMED", "sim/cockpit2/autopilot/altitude_hold_armed") dataref("AP_STATE", "sim/cockpit/autopilot/autopilot_state") dataref("xp_autopilot_mode", "sim/cockpit/autopilot/autopilot_mode", "writable") dataref("xp_autopilot_airspeed", "sim/cockpit/autopilot/airspeed", "writable") dataref("xp_autothrottle_enabled", "sim/cockpit2/autopilot/autothrottle_enabled", "writable") dataref("QNH_Pilot", "sim/cockpit2/gauges/actuators/barometer_setting_in_hg_pilot", "writable") -- define a global variable to be used in other scripts (shared with another script) autopilot_helper_vvi = 600 -- init the HUD HUD.begin_HUD( -81, 1, 80, 315, "my_radios", "always") -- Autopilot HUD.create_element("AP", 0, 280, 40, 35) HUD.draw_string(5, 10, 18, "AP") HUD.create_backlight_indicator( 30, 0, 10, 35, "xp_autopilot_mode == 1", 1, 1, 0, 1) HUD.create_backlight_indicator( 30, 0, 10, 35, "xp_autopilot_mode == 2", 0, 1, 0, 1) HUD.create_click_switch(0, 0, 40, 35, "xp_autopilot_mode", 1, 2) -- Speed HUD.create_element("Speed", 40, 280, 40, 35) HUD.draw_string(5, 23, 10, "SPD") HUD.draw_fstring(5, 5, 18, "%03i", "xp_autopilot_airspeed") HUD.create_wheel_action(0, 0, 25, 35, "xp_autopilot_airspeed = math.floor(xp_autopilot_airspeed / 5 + MOUSE_WHEEL_CLICKS) * 5") HUD.create_wheel_action(25, 0, 15, 35, "xp_autopilot_airspeed = xp_autopilot_airspeed + MOUSE_WHEEL_CLICKS") HUD.create_backlight_indicator( 30, 0, 10, 35, "xp_autothrottle_enabled == 1", 0, 1, 0, 1) HUD.create_click_switch(0, 0, 40, 35, "xp_autothrottle_enabled", 0, 1) -- ALT HUD.create_element("ALT", 0, 245, 80, 35) HUD.draw_string(5, 23, 10, "ALT") HUD.draw_fstring(15, 5, 18, "%05i", "ALT") HUD.create_wheel_action( 0, 0, 35, 35, "ALT = math.floor(ALT / 100 + MOUSE_WHEEL_CLICKS * 10) * 100") HUD.create_wheel_action(36, 0, 40, 35, "ALT = math.floor(ALT / 100 + MOUSE_WHEEL_CLICKS) * 100") HUD.create_wheel_action( 0, 0, 80, 35, "autopilot_helper_set_VVI()") HUD.create_backlight_indicator( 70, 0, 10, 35, "bit.band(AP_STATE, 32) > 0", 1, 0, 0, 1) HUD.create_backlight_indicator( 70, 0, 10, 35, "bit.band(AP_STATE, 16384) > 0", 0, 1, 0, 1) HUD.create_click_action(0, 0, 80, 35, 'command_once("sim/autopilot/altitude_hold")') -- VVI HUD.create_element("VVI", 0, 210, 80, 35) HUD.draw_string(5, 23, 10, "V V I") HUD.draw_fstring(25, 5, 18, "%04i", "VVI") HUD.create_wheel_action(0, 0, 80, 35, "VVI = math.floor(VVI / 100 + MOUSE_WHEEL_CLICKS) * 100") HUD.create_backlight_indicator( 70, 0, 10, 35, "bit.band(AP_STATE, 16) > 0", 0, 1, 0, 1) HUD.create_click_action(0, 0, 80, 35, 'command_once("sim/autopilot/vertical_speed")') -- COM1 radio HUD.create_element("COM", 0, 140, 80, 35) HUD.draw_string(5, 23, 10, "COM") HUD.draw_fstring(12, 5, 18, "%3.2f", "COM1/100") HUD.create_wheel_action( 0, 0, 45, 35, "COM1 = COM1 + MOUSE_WHEEL_CLICKS * 100") HUD.create_wheel_action(45, 0, 10, 35, "COM1 = COM1 + MOUSE_WHEEL_CLICKS * 10") HUD.create_wheel_action(55, 0, 25, 35, "COM1 = COM1 + MOUSE_WHEEL_CLICKS") -- UNICOM HUD.create_click_action(0, 0, 80, 35, "COM1 = 12280") -- NAV1 radio HUD.create_element("NAV", 0, 105, 80, 35) HUD.draw_string(5, 23, 10, "NAV") HUD.draw_fstring(12, 5, 18, "%3.2f", "NAV1/100") HUD.create_wheel_action( 0, 0, 45, 35, "NAV1 = NAV1 + MOUSE_WHEEL_CLICKS * 100") HUD.create_wheel_action(45, 0, 10, 35, "NAV1 = NAV1 + MOUSE_WHEEL_CLICKS * 10") HUD.create_wheel_action(55, 0, 25, 35, "NAV1 = NAV1 + MOUSE_WHEEL_CLICKS") HUD.create_backlight_indicator( 70, 0, 10, 35, "bit.band(AP_STATE, 256) > 0", 1, 0, 0, 1) HUD.create_backlight_indicator( 70, 0, 10, 35, "bit.band(AP_STATE, 512) > 0", 0, 1, 0, 1) HUD.create_click_action(0, 0, 80, 35, 'command_once("sim/autopilot/NAV")') -- ADF1 radio HUD.create_element("ADF", 0, 70, 80, 35) HUD.draw_string(5, 23, 10, "ADF") HUD.draw_fstring(27, 5, 18, "%04i", "ADF1") HUD.create_wheel_action( 0, 0, 45, 35, "ADF1 = ADF1 + MOUSE_WHEEL_CLICKS * 100") HUD.create_wheel_action(45, 0, 10, 35, "ADF1 = ADF1 + MOUSE_WHEEL_CLICKS * 10") HUD.create_wheel_action(55, 0, 25, 35, "ADF1 = ADF1 + MOUSE_WHEEL_CLICKS") -- OBS1 HUD.create_element("OBS", 40, 175, 40, 35) HUD.draw_string(5, 23, 10, "OBS") HUD.draw_fstring(5, 5, 18, "%03i", "OBS1") HUD.create_wheel_action(0, 0, 25, 35, "OBS1 = math.floor(OBS1 / 10 + MOUSE_WHEEL_CLICKS) * 10") HUD.create_wheel_action(25, 0, 15, 35, "OBS1 = OBS1 + MOUSE_WHEEL_CLICKS") HUD.create_click_action(0, 0, 40, 35, 'command_once("sim/autopilot/NAV")') -- HDG HUD.create_element("HDG", 0, 175, 40, 35) HUD.draw_string(5, 23, 10, "HDG") HUD.draw_fstring(5, 5, 18, "%03i", "HDG") HUD.create_wheel_action(0, 0, 25, 35, "HDG = math.floor(HDG / 10 + MOUSE_WHEEL_CLICKS) * 10") HUD.create_wheel_action(25, 0, 15, 35, "HDG = HDG + MOUSE_WHEEL_CLICKS") HUD.create_backlight_indicator( 30, 0, 10, 35, "bit.band(AP_STATE, 2) > 0", 0, 1, 0, 1) HUD.create_click_action(0, 0, 40, 35, 'command_once("sim/autopilot/heading")') -- SQUAWK HUD.create_element("SQUAWK", 0, 35, 80, 35) HUD.draw_string(5, 23, 10, "SQUAWK") HUD.draw_fstring(27, 5, 18, "%04i", "SQUAWK") HUD.create_wheel_action( 27, 0, 9, 35, "SQUAWK = SQUAWK + 1000 * MOUSE_WHEEL_CLICKS") HUD.create_wheel_action( 37, 0, 9, 35, "SQUAWK = SQUAWK + 100 * MOUSE_WHEEL_CLICKS") HUD.create_wheel_action( 47, 0, 9, 35, "SQUAWK = SQUAWK + 10 * MOUSE_WHEEL_CLICKS") HUD.create_wheel_action( 57, 0, 9, 35, "SQUAWK = SQUAWK + MOUSE_WHEEL_CLICKS") HUD.create_click_switch( 0, 0, 80, 35, "TRANSPONDER_MODE", 1, 2) HUD.create_backlight_indicator( 70, 0, 10, 35, "TRANSPONDER_MODE == 0", 0, 0, 1, 1) HUD.create_backlight_indicator( 70, 0, 10, 35, "TRANSPONDER_MODE == 1", 1, 0, 0, 1) HUD.create_backlight_indicator( 70, 0, 10, 35, "TRANSPONDER_MODE == 2", 0, 1, 0, 1) HUD.create_backlight_indicator( 70, 0, 10, 35, "TRANSPONDER_MODE == 3", 1, 1, 0, 1) -- BARO HUD.create_element("BARO", 0, 0, 80, 35) HUD.draw_string(5, 23, 10, "BARO") if XPLANE_LANGUAGE == "English" then HUD.draw_fstring(25, 5, 18, "%2.2f", "QNH_Pilot") else HUD.draw_fstring(25, 5, 18, "%4.0f", "QNH_Pilot * 33.86531") end HUD.create_wheel_action(0, 0, 80, 35, "QNH_Pilot = QNH_Pilot + MOUSE_WHEEL_CLICKS / 100") HUD.create_click_action(0, 0, 80, 35, 'QNH_Pilot = 29.92') -- finish the HUD HUD.end_HUD() -- define a function uesed by the HUD function autopilot_helper_set_VVI() if ALT > ELEVATION*3.2808399 then if bit.band(AP_STATE, 16) == 0 then command_once( "sim/autopilot/vertical_speed" ) end VVI = autopilot_helper_vvi if bit.band(AP_STATE, 32) == 0 then command_once("sim/autopilot/altitude_arm") end end if ALT < ELEVATION*3.2808399 then if bit.band(AP_STATE, 16) == 0 then command_once( "sim/autopilot/vertical_speed" ) end VVI = -autopilot_helper_vvi if bit.band(AP_STATE, 32) == 0 then command_once("sim/autopilot/altitude_arm") end end end -- define some functions taken from another script plane_PSI = dataref_table("sim/flightmodel/position/psi") autopilot_mode = dataref_table("sim/cockpit/autopilot/autopilot_mode") function activate_autopilot_tweak() HDG = plane_PSI[0] command_once( "sim/autopilot/reentry" ) command_once( "sim/autopilot/heading" ) command_once( "sim/autopilot/altitude_hold" ) ALT = ELEVATION*3.2808399 autopilot_mode[0] = 2 end function stop_autopilot_tweak() autopilot_mode[0] = 0 xp_autothrottle_enabled = 0 end -- provide a custom command to activate it create_command( "FlyWithLua/autopilot/activate_autopilot", "activate autopilot and set actual heading and altitude", "activate_autopilot_tweak()", "", "" ) create_command( "FlyWithLua/autopilot/set_autopilot_off", "deactivate autopilot tweak and get back normal steering", "stop_autopilot_tweak()", "", "" ) -- do some corrections function radio_limiter() if COM1 < 11800 then COM1 = 11800 end if COM1 > 13697 then COM1 = 13697 end if NAV1 < 10800 then NAV1 = 10800 end if NAV1 > 11797 then NAV1 = 11797 end if ADF1 < 530 then ADF1 = 530 end if ADF1 > 1700 then ADF1 = 1700 end if OBS1 < 0 then OBS1 = 350 end if OBS1 > 360 then OBS1 = 10 end if HDG < 0 then HDG = 350 end if HDG > 360 then HDG = 10 end local SQUAWKSTRING = string.format("%4i", SQUAWK) SQUAWKSTRING = string.gsub(SQUAWKSTRING, '8', '0') SQUAWKSTRING = string.gsub(SQUAWKSTRING, '9', '0') SQUAWK = tonumber(SQUAWKSTRING) if SQUAWK == 7500 then SQUAWK = 7550 end if SQUAWK < 0 then SQUAWK = 7000 end end do_on_mouse_wheel("radio_limiter()")
画面の右下にマウスを持っていくと表示される。クリックやマウスホイールが使える
フローティングウインドウfloat_wnd_set_ondraw()の場合
関数では必ずこのようになる「function gps2w_ondraw(test_wnd, x, y)」太文字のところが必要になる。test_wndでウインドウを指定、xとyでそのウインドウの位置を指定することができる。
従ってクリック関数においても必ず位置関係が必要になる場合はこの(test_wnd, x, y)が付いてくることになる。
そして大事なことはクリックの場合、FlyWithLuaの一般的なクリック関数が使えない。
例えば、MOUSE_STATUS == “down”とかMOUSE_STATUS == “drag”等は使えない。
それとこれが必要、クリック関数「unction gps2w_onclick(test_wnd, x, y, state)」このように最後にstate(マウスダウン)を入れる必要がある。これをマウスダウンに使う。これは変数なので名前は何でも良い。
require("graphics") -- datarefs -- local mag_var = dataref_table("sim/flightmodel/position/magnetic_variation") -- float n degrees local magnetic variation local test_wnd = nil -- window handle local winLeft, winTop, winRight, winBottom = 0,0,0,0 -- float_wnd_get_geometry local dWidth, dHeight = 0,0 -- float_wnd_get_dimensions -- functions ------------------------------------------------------------------ function gps2w_ondraw(test_wnd, x, y) dWidth, dHeight = float_wnd_get_dimensions(test_wnd) winLeft, winTop, winRight, winBottom = float_wnd_get_geometry(test_wnd) -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- draw box around window XPLMSetGraphicsState(0,0,0,1,1,0,0) graphics.set_color(1,1,1,0.3) graphics.draw_rectangle(x+18, y+205, x+75, y+220) --長方形を描く draw_string(winLeft+20,winTop-152, "Q-Search","red") end -- function gps2w_ondraw function gps2w_onclick(test_wnd, x, y, state) -- ボタンダウンアクション if (state == 1) then if (x >= 18 and x <= 75 and y >= 205 and y <= 220) then--865 877 command_once("sim/flight_controls/flaps_up") end end -- if state end -- function gps2w_onclick -- window close function gps2w_onclose(test_wnd) float_wnd_destroy(test_wnd) end test_wnd = float_wnd_create(171, 362, 1, false) float_wnd_set_title(test_wnd, "gps2w_xp11") float_wnd_set_position(test_wnd, 180, 150) float_wnd_set_ondraw(test_wnd, "gps2w_ondraw") float_wnd_set_onclick(test_wnd, "gps2w_onclick") float_wnd_set_onclose(test_wnd, "gps2w_onclose")
float_wnd_set_imgui_builder()の場合
ボタンのデフォルトと押したときの色を変える
imgui.Button(およびimgui.ImageButton)の色は、次の3つの方法で制御できる。
imgui.PushStyleColor(imgui.constant.Col.Button, 0x00404040 )–デフォルト状態
imgui.PushStyleColor(imgui.constant.Col.ButtonHovered, 0x40404040)–マウスが乗った状態
imgui.PushStyleColor(imgui.constant.Col.ButtonActive, 0x80404040)–マウスクリック時の状態
最後のButtonActiveはマウスでクリックした瞬間に色が変化する。
さらに、ボタンのテキストの色は、以下の方法で設定できる。「x」の後の最初の2桁には、アルファチャンネルまたは透明度の値が含まれている。(それが完全に正確かどうかはわかりません。)0x00で始まるこれらの値は、完全に透過的であるため、表示から隠されて見えなくなる。
imgui.PushStyleColor(imgui.constant.Col.Text, 0x00FFFFFF)
command_once–一度だけ
command_begin–beginとは始める(始まり)その後も続く
command_end--止める
imgui.IsItemActive()
ボタントグル
ボタンを押すとテキストの色がグリーンから赤色に変わる。更にボタンを押すと交互に色が変わるというトグルスイッチになる。
if not SUPPORTS_FLOATING_WINDOWS then -- to make sure the script doesn't stop old FlyWithLua versions logMsg("imgui not supported by your FlyWithLua version") return end -- imgui only works inside a floating window, so we need to create one first: demo_wnd = float_wnd_create(100, 150, 1, true) float_wnd_set_title(demo_wnd, "imgui Demo") float_wnd_set_imgui_builder(demo_wnd, "build_demo") float_wnd_set_onclose(demo_wnd, "closed_demo") --ポジションが抜けている makeRed = false --初期は赤色でない text = "" function build_demo(wnd, x, y) -- 次の関数はボタンを作成します。 if imgui.Button("Push Me") then -- Button関数は、指定されたラベルでボタンを作成します。 ボタンが離された瞬間にtrueを返します。 --つまり、ifステートメントを使用して、ボタンの背後にあるアクションを実行する必要があるかどうかを確認します。 --ボタンを変数に切り替えましょう: makeRed = not makeRed --falseの否定になるのでtrueを返す(trueとfalseを繰り返す) end -- 変数を使用してテキストの色を変更します if makeRed then --makeRedはtrueなので赤を表示 imgui.PushStyleColor(imgui.constant.Col.Text, 0xFF0000FF)--レッド(最初にボタンを押したらこの色に変化) else imgui.PushStyleColor(imgui.constant.Col.Text, 0xFF00FF00)--ライトグリーン(ボタンを押さない最初はこの色) end imgui.TextUnformatted("Some Text") imgui.PopStyleColor() end
マウスの設定でスライダーの動きをコントロール
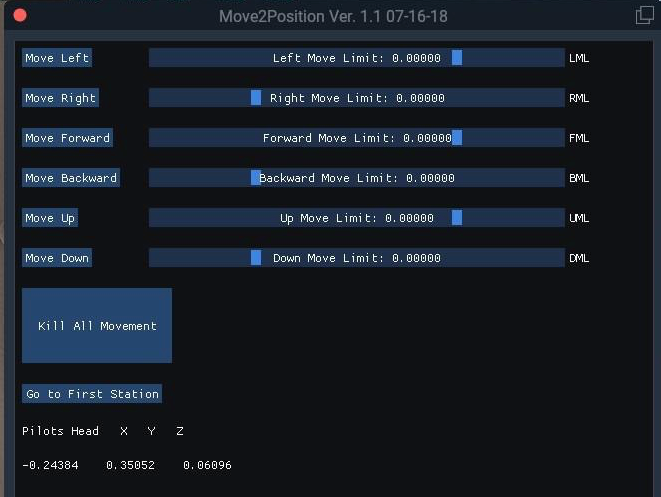
-- Move2Position Window -- William R. Good Ver. 1.1 07-16-18 if not SUPPORTS_FLOATING_WINDOWS then -- to make sure the script doesn't stop old FlyWithLua versions logMsg("imgui not supported by your FlyWithLua version") return end dataref("PilotHeadX_mtp", "sim/graphics/view/pilots_head_x", "readonly") dataref("PilotHeadY_mtp", "sim/graphics/view/pilots_head_y", "readonly") dataref("PilotHeadZ_mtp", "sim/graphics/view/pilots_head_z", "readonly") dataref("PilotHeadPSI_mtp", "sim/graphics/view/pilots_head_psi", "readonly") dataref("PilotHeadTHE_mtp", "sim/graphics/view/pilots_head_the", "readonly") dataref("PilotHeadPHI_mtp", "sim/graphics/view/pilots_head_phi", "readonly") mtp_continue1 = 0 mtp_continue2 = 0 mtp_continue3 = 0 mtp_continue4 = 0 mtp_continue5 = 0 mtp_continue6 = 0 mtp_continue7 = 0 mtp_continue8 = 0 left_move_continue = 0 right_move_continue = 0 forward_move_continue = 0 backward_move_continue = 0 up_move_continue = 0 down_move_continue = 0 station1_step_number = 0 station2_step_number = 0 station3_step_number = 0 station4_step_number = 0 station5_step_number = 0 left_limit = 0 right_limit = 0 forward_limit = 0 backward_limit = 0 up_limit = 0 down_limit = 0 mtp_loop = 0 function mtp_on_build(mtp_wnd, x, y) imgui.Button("Move Left") if imgui.IsItemActive() then if PilotHeadX_mtp > left_limit then if mtp_continue1 < 1 then command_begin("sim/general/left_fast") mtp_continue1 = 1 end end end imgui.SameLine() imgui.TextUnformatted(" ") imgui.SameLine() -- The following function creates a slider: local changed, newVal = imgui.SliderFloat("LML", left_limit, -30, 10, "Left Move Limit: %.5f") if changed then left_limit = newVal end imgui.TextUnformatted("") imgui.Button("Move Right") if imgui.IsItemActive() then if PilotHeadX_mtp < right_limit then if mtp_continue2 < 1 then command_begin("sim/general/right_fast") mtp_continue2 = 1 end end end imgui.SameLine() imgui.TextUnformatted(" ") imgui.SameLine() -- The following function creates a slider: local changed, newVal = imgui.SliderFloat("RML", right_limit, -10, 30, "Right Move Limit: %.5f") if changed then right_limit = newVal end imgui.TextUnformatted("") imgui.Button("Move Forward") if imgui.IsItemActive() then if PilotHeadZ_mtp > forward_limit then if mtp_continue3 < 1 then command_begin("sim/general/forward_fast") mtp_continue3 = 1 end end end imgui.SameLine() imgui.TextUnformatted(" ") imgui.SameLine() -- The following function creates a slider: local changed, newVal = imgui.SliderFloat("FML", forward_limit, -30, 10, "Forward Move Limit: %.5f") if changed then forward_limit = newVal end imgui.TextUnformatted("") imgui.Button("Move Backward") if imgui.IsItemActive() then if PilotHeadZ_mtp < backward_limit then if mtp_continue4 < 1 then command_begin("sim/general/backward_fast") mtp_continue4 = 1 end end end imgui.SameLine() imgui.TextUnformatted(" ") imgui.SameLine() -- The following function creates a slider: local changed, newVal = imgui.SliderFloat("BML", backward_limit, -10, 30, "Backward Move Limit: %.5f") if changed then backward_limit = newVal end imgui.TextUnformatted("") imgui.Button("Move Up") if imgui.IsItemActive() then if PilotHeadY_mtp < up_limit then if mtp_continue5 < 1 then command_begin("sim/general/up_fast") mtp_continue5 = 1 end end end imgui.SameLine() imgui.TextUnformatted(" ") imgui.SameLine() -- The following function creates a slider: local changed, newVal = imgui.SliderFloat("UML", up_limit, -30, 10, "Up Move Limit: %.5f") if changed then up_limit = newVal end imgui.TextUnformatted("") imgui.Button("Move Down") if imgui.IsItemActive() then if PilotHeadY_mtp > down_limit then if mtp_continue6 < 1 then command_begin("sim/general/down_fast") mtp_continue6 = 1 end end end imgui.SameLine() imgui.TextUnformatted(" ") imgui.SameLine() -- The following function creates a slider: local changed, newVal = imgui.SliderFloat("DML", down_limit, -10, 30, "Down Move Limit: %.5f") if changed then down_limit = newVal end imgui.TextUnformatted("") imgui.Button("Kill All Movement", 150, 75) if imgui.IsItemActive() then mtp_continue1 = 0 mtp_continue2 = 0 mtp_continue3 = 0 mtp_continue4 = 0 mtp_continue5 = 0 mtp_continue6 = 0 mtp_continue7 = 0 mtp_continue8 = 0 left_move_continue = 0 right_move_continue = 0 forward_move_continue = 0 backward_move_continue = 0 up_move_continue = 0 down_move_continue = 0 station1_step_number = 0 station2_step_number = 0 station3_step_number = 0 station4_step_number = 0 station5_step_number = 0 command_end("sim/general/left_fast") command_end("sim/general/right_fast") command_end("sim/general/forward_fast") command_end("sim/general/backward_fast") command_end("sim/general/up_fast") command_end("sim/general/down_fast") command_once("sim/flight_controls/door_close_1") end imgui.TextUnformatted("") -- First test of my walk around preflight imgui.Button("Go to First Station") if imgui.IsItemActive() then first_station = 1 station1_step_number = 1 end if first_station == 1 then if station1_step_number == 1 then command_once("sim/flight_controls/door_open_1") if mtp_loop < 100 then mtp_loop = mtp_loop + 1 if mtp_loop == 90 then mtp_loop = 0 station1_step_number = 2 end end end if station1_step_number == 2 then if PilotHeadX_mtp > - 2.0 then if left_move_continue < 1 then command_begin("sim/general/left_fast") left_move_continue = 1 end else left_move_continue = 0 command_end("sim/general/left_fast") if mtp_loop < 100 then mtp_loop = mtp_loop + 1 if mtp_loop == 90 then mtp_loop = 0 station1_step_number = 3 end end end end if station1_step_number == 3 then if PilotHeadY_mtp > 0.0 then if down_move_continue < 1 then command_begin("sim/general/down_fast") down_move_continue = 1 end else down_move_continue = 0 command_end("sim/general/down_fast") if mtp_loop < 100 then mtp_loop = mtp_loop + 1 if mtp_loop == 90 then mtp_loop = 0 station1_step_number = 4 end end end end if station1_step_number == 4 then if PilotHeadZ_mtp < 5.5 then if backward_move_continue < 1 then command_begin("sim/general/backward_fast") backward_move_continue = 1 end else backward_move_continue = 0 command_end("sim/general/backward_fast") if mtp_loop < 100 then mtp_loop = mtp_loop + 1 if mtp_loop == 90 then mtp_loop = 0 station1_step_number = 5 end end end end if station1_step_number == 5 then if PilotHeadX_mtp < - 1.34 then if right_move_continue < 1 then command_begin("sim/general/right_fast") right_move_continue = 1 end else right_move_continue = 0 command_end("sim/general/right_fast") first_station = 0 station1_step_number = 0 end end end imgui.TextUnformatted("") imgui.TextUnformatted("Pilots Head X Y Z") imgui.TextUnformatted("") PilotHeadX_mtpRound = tonumber(string.format("%.5f", PilotHeadX_mtp)) imgui.TextUnformatted(PilotHeadX_mtpRound) imgui.SameLine() imgui.TextUnformatted(" ") imgui.SameLine() PilotHeadY_mtpRound = tonumber(string.format("%.5f", PilotHeadY_mtp)) imgui.TextUnformatted(PilotHeadY_mtpRound) imgui.SameLine() imgui.TextUnformatted(" ") imgui.SameLine() PilotHeadZ_mtpRound = tonumber(string.format("%.5f", PilotHeadZ_mtp)) imgui.TextUnformatted(PilotHeadZ_mtpRound) if imgui.IsMouseReleased then if mtp_continue1 == 1 then command_end("sim/general/left_fast") mtp_continue1 = 0 end if mtp_continue2 == 1 then command_end("sim/general/right_fast") mtp_continue2 = 0 end if mtp_continue3 == 1 then command_end("sim/general/forward_fast") mtp_continue3 = 0 end if mtp_continue4 == 1 then command_end("sim/general/backward_fast") mtp_continue4 = 0 end if mtp_continue5 == 1 then command_end("sim/general/up_fast") mtp_continue5 = 0 end if mtp_continue6 == 1 then command_end("sim/general/down_fast") mtp_continue6 = 0 end mtp_continue1 = 0 mtp_continue2 = 0 mtp_continue3 = 0 mtp_continue4 = 0 mtp_continue5 = 0 mtp_continue6 = 0 mtp_continue7 = 0 mtp_continue8 = 0 end end mtp_wnd = nil function mtp_show_wnd() mtp_wnd = float_wnd_create(640, 480, 1, true) float_wnd_set_title(mtp_wnd, "Move2Position Ver. 1.1 07-16-18") float_wnd_set_imgui_builder(mtp_wnd, "mtp_on_build") end function mtp_hide_wnd() if mtp_wnd then float_wnd_destroy(mtp_wnd) end end mtp_show_only_once = 0 mtp_hide_only_once = 0 function toggle_move2position() mtp_show_window = not mtp_show_window if mtp_show_window then if mtp_show_only_once == 0 then mtp_show_wnd() mtp_show_only_once = 1 mtp_hide_only_once = 0 end else if mtp_hide_only_once == 0 then mtp_hide_wnd() mtp_hide_only_once = 1 mtp_show_only_once = 0 end end end add_macro("Move2Position: open/close", "mtp_show_wnd()", "mtp_hide_wnd()", "deactivate") create_command("FlyWithLua/move2position/show_toggle", "open/close move2position", "toggle_move2position()", "", "")